Routing Status Signal¶
In this guide you will learn how to use RoutingService
to compute a route and receive status notification signals from the
routing service when connected or disconnected from the internet,
which helps troubleshoot issues.
Receive routing status signals¶
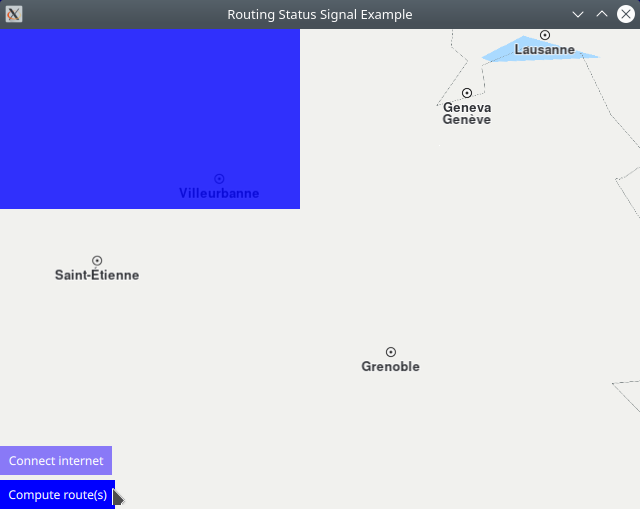
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
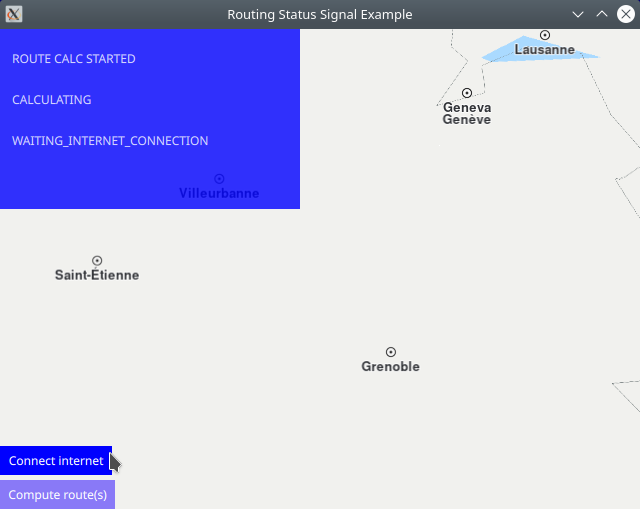
RoutingStatusSignal
demonstrates using RoutingService
to
calculate a route between preset start (departure) and end (destination) points,
with 0 or more optional waypoints in between, 1 in this case.
Notification signals received from the routing service are displayed on screen
in a scrollable list in the blue panel at the top left.
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the RoutingStatusSignal example folder and open RoutingStatusSignal.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
The cache directory for this example should be deleted first; for example, on linux, the full path would be:
/home/username/.cache/RoutingStatusSignal/
In main.qml, we import the GeneralMagic QML plugin. Next, we set
ServicesManager.settings.allowInternetConnection = false
,
which means there is no network connection, and thus no maps can be downloaded,
so that we can test the notification signals indicating that a route cannot be calculated,
as we just deleted the cache, above, to make sure there are no offline maps.
1import QtQuick 2.12
2import QtQuick.Controls 2.12
3import QtQuick.Layouts 1.12
4//! [Routing wiring]
5import QtQuick.Window 2.12
6import GeneralMagic 2.0
7
8Window {
9 visible: true
10 width: 640
11 height: 480
12 title: qsTr("Routing Status Signal Example")
13
14 property var updater: ServicesManager.contentUpdater(ContentItem.Type.RoadMap)
15 Component.onCompleted: {
16 //! [Set token safely]
17 ServicesManager.settings.token = __my_secret_token;
18 //! [Set token safely]
19 ServicesManager.settings.allowInternetConnection = false; // enable connection to online services
20
21 updater.autoApplyWhenReady = true;
22 updater.update();
23 }
Compute the route(s)¶
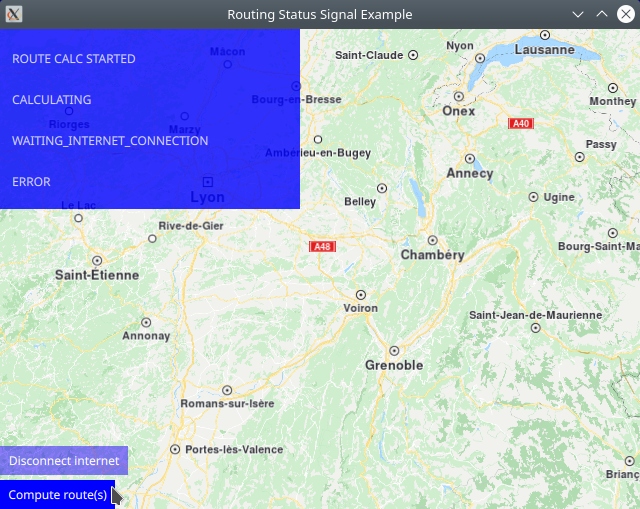
The first step is to declare a RoutingService
to be used for calculating the route(s) between preset
start (departure) and end (destination) points,
with 0 or more optional waypoints in between, 1 in this case,
which are specified as a LandmarkList
1RoutingService {
2 id: routingService
3 type: Route.Type.Fastest
4 transportMode: Route.TransportMode.Car
5
6 waypoints: LandmarkList {
7 Landmark {
8 name: "start"
9 coordinates: Coordinates {
10 latitude: 41.08769
11 longitude: 1.15759
12 }
13 }
14 Landmark {
15 name: "waypoint1"
16 coordinates: Coordinates {
17 latitude: 42.53584
18 longitude: 1.52782
19 }
20 }
21 Landmark {
22 name: "stop"
23 coordinates: Coordinates {
24 latitude: 42.53505
25 longitude: 3.04153
26 }
27 }
28 }
29 onStarted: {
30 messageModel.append({message: "ROUTE CALC STARTED"});
31 console.log("~~~ RoutingService.onStarted called: hasProgress:" + hasProgress);
32 }
33
34 onProgress: {
35 messageModel.append({message: "ROUTE CALC PROGRESS"});
36 console.log("~~~ RoutingService.onProgress called: percentage:" + percentage);
37 }
38 onStatusChanged: {
39 switch ( status )
40 {
41 case 0:
42 messageModel.append({message: "UNINITIALIZED"});
43 console.log("~~~ RoutingService.onStatusChanged called: status UNINITIALIZED"); break;
44 case 1:
45 messageModel.append({message: "CALCULATING"});
46 console.log("~~~ RoutingService.onStatusChanged called: status CALCULATING"); break;
47 case 2:
48 messageModel.append({message: "WAITING_INTERNET_CONNECTION"});
49 console.log("~~~ RoutingService.onStatusChanged called: status WAITING_INTERNET_CONNECTION"); break;
50 case 3:
51 messageModel.append({message: "READY"});
52 console.log("~~~ RoutingService.onStatusChanged called: status READY"); break;
53 case 4:
54 messageModel.append({message: "ERROR"});
55 console.log("~~~ RoutingService.onStatusChanged called: status ERROR"); break;
56 default:
57 messageModel.append({message: "UNDEFINED"});
58 console.log("~~~ RoutingService.onStatusChanged called: status UNDEFINED"); break;
59 }
60 }
61 onFinished: {
62 messageModel.append({message: "ROUTE CALC FINISHED"});
63 console.log("~~~ RoutingService.onFinished called: Result:" + result + "; hint:" + hint);
64 mapView.routeCollection.set(routeList);
65 mapView.centerOnRouteList(routeList);
66 }
67}
The RoutingService
signals indicating the state of the route calculation are implemented:
onStarted:
,
onProgress:
,
onStatusChanged:
, containing 6 possible states, and
onFinished:
so when they are received, a log message is printed in the application output window in the IDE, and a short message is added to a scrollable list which is displayed in the qml GUI in the top left blue panel.
Display routing status signals¶
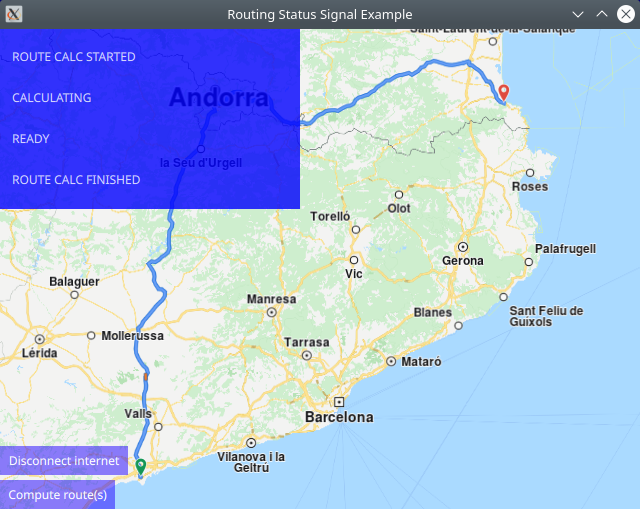
An interactive map is displayed using MapView
and automatically centered on
the calculated route, if any, as soon as it is selected.
1MapView {
2 id: mapView
3 anchors.fill: parent
4 viewAngle: 25
5 cursorVisibility: false
6
7 onRouteSelected: {
8 routeCollection.mainRoute = route;
9 centerOnRoute(route);
10 console.log("Route selected, centering:" + route.summary);
11 }
12
13 ListModel {
14 id: messageModel
15 }
16 Rectangle {
17 height: 180
18 width: 300
19 opacity: 0.8
20 color: "#0000ff"
21 anchors.left: parent.left
22 anchors.top: parent.top
23 ColumnLayout {
24 anchors { fill: parent }
25 ListView {
26 Layout.fillWidth: true
27 Layout.maximumHeight: 0.9 * parent.height
28 Layout.fillHeight: true
29 model: messageModel
30 delegate: ItemDelegate {
31 text: model.message
32 Binding {
33 target: contentItem
34 property: "color"
35 value: highlighted ? "#00ff00" : "#ffffff"
36 }
37 }
38 }
39 }
40 }
The ListModel
and the Rectangle
element containing the scrollable ListView
implement the blue panel at the top left of the viewport where the routing status signals
received are dynamically added to the scrollable list to be displayed.
1ColumnLayout {
2 anchors.left: parent.left
3 anchors.bottom: parent.bottom
4 Button {
5 text: ServicesManager.settings.allowInternetConnection ? "Disconnect internet" : "Connect internet"
6 background: Rectangle {
7 opacity: parent.hovered ? 1 : 0.5
8 color: enabled ? parent.down ? "#aa00aa" :
9 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
10 }
11 palette { buttonText: "#ffffff"; }
12 onClicked:
13 // enable connection to online services
14 ServicesManager.settings.allowInternetConnection = !ServicesManager.settings.allowInternetConnection;
15 }
16 Button {
17 text: "Compute route(s)"
18 //enabled: ServicesManager.settings.connected
19 background: Rectangle {
20 opacity: parent.hovered ? 1 : 0.5
21 color: enabled ? parent.down ? "#aa00aa" :
22 (parent.hovered ? "#0000ff" : "#2000ff") : "#aaaaaa"
23 }
24 palette { buttonText: "#ffffff"; }
25 onClicked: routingService.update()
26 }
27}
At the bottom left, a convenience button is added to connect/disconnect the internet, which affects whether maps can be downloaded, and a button to compute a route for the preselected list of waypoints.