Markers Programmatic¶
In this guide you will learn how to programmatically add markers shown as icons with a custom image at specified coordinates on the map. Additional custom icon images can be specified for three density levels of marker groupings at more distant zoom levels.
Add custom icons programmatically¶
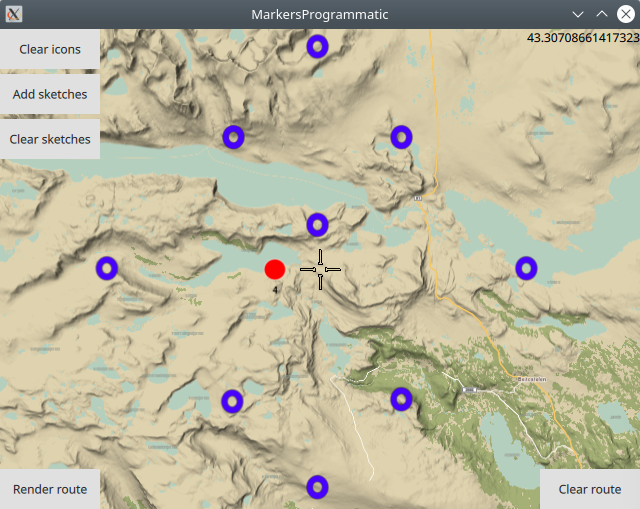
First, get an API key token, see the Getting Started guide.
Qt should be installed to continue.The Maps SDK for Qt should be installed, see the Setup Maps SDK for Qt guide.
Overview¶
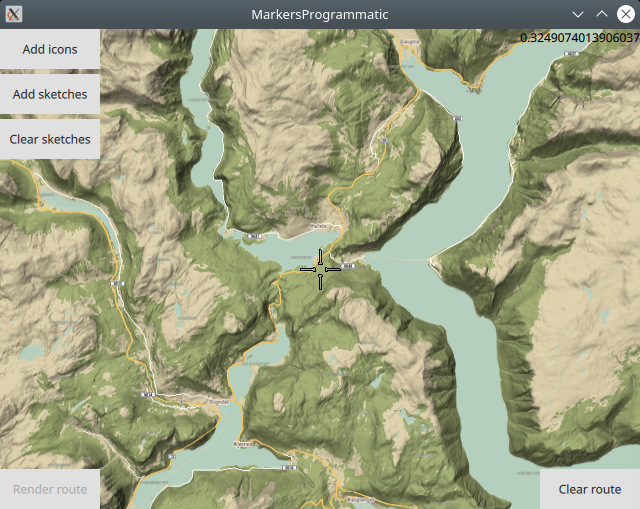
MarkersProgrammatic
demonstrates how easy it is to use l MapView
to display an interactive map, and add markers programmatically at
specified coordinates, which are displayed as icons on the map.
Optionally, the marker icons can be grouped upon zooming out.
Also, the markers can be used as waypoints for rendering a route.
There are two types of markers: sketches-type markers and regular markers.
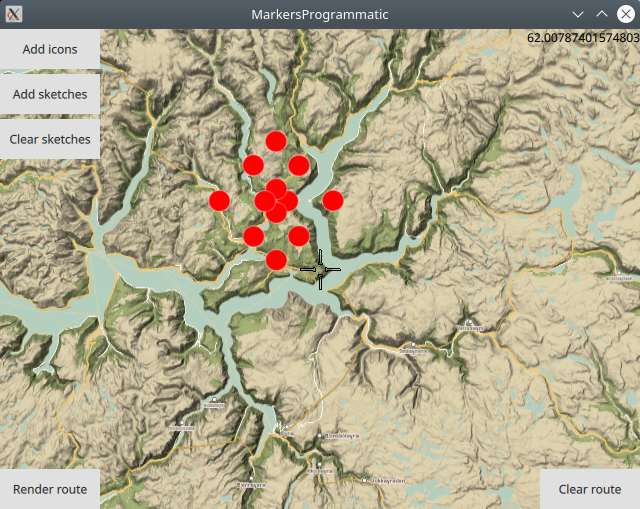
The sketches-type markers cannot be grouped, and all of them are displayed regardless of the zoom level. A custom icon image can be configured for rendering the icons. If no image is configured, a blue filled circle will be used as the icon.
Setting the custom icon image for sketches-type markers - see qml.qrc and main.qml:
markerRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/redcircle.png")
Alternatively, instead of using a custom icon image, sketches-type markers can also be rendered on the map using the predefined red- green- or blue- filled circles, specified by enum value - see main.qml:
markerRenderSettings.icon = ServicesManager.createIconFromId(Icon.GreenBall);
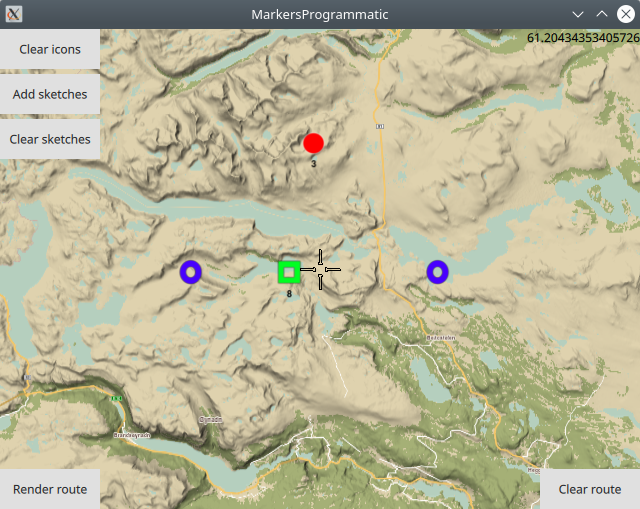
The regular markers support grouping in 3 user-specified density levels. In addition to the custom image used for rendering single marker icons, a separate custom icon image can be configured for each of the 3 marker grouping levels: low-, medium- and high- density, see qml.qrc and main.qml:
1markerListRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/violetcircle.png");
2markerListRenderSettings.lowDensityPointsGroupIcon = ServicesManager.createIconFromFile("qrc:/redcircle.png");
3markerListRenderSettings.mediumDensityPointsGroupIcon = ServicesManager.createIconFromFile("qrc:/greensquare.png");
4markerListRenderSettings.highDensityPointsGroupIcon = ServicesManager.createIconFromFile("qrc:/bluetriangle.png");
The custom icon images, such as png images, optionally with transparency, and map styles must be located in the project directory and configured in the qml.qrc file, shown below:
1<RCC>
2 <qresource prefix="/">
3 <file>main.qml</file>
4 <file>redcircle.png</file>
5 <file>greensquare.png</file>
6 <file>bluetriangle.png</file>
7 <file>violetcircle.png</file>
8 <file>Basic_1_Night_Blues_with_Elevation-1_5_688.style</file>
9 <file>Basic_1_Oldtime_with_Elevation-1_12_657.style</file>
10 </qresource>
11</RCC>
Alternatively, instead of using custom icon images, regular markers can also be rendered on the map using the predefined red- green- or blue- filled circles, specified by enum value - see main.qml:
1markerListRenderSettings.lowDensityPointsGroupIcon = ServicesManager.createIconFromId(Icon.RedBall);
2markerListRenderSettings.mediumDensityPointsGroupIcon = ServicesManager.createIconFromId(Icon.GreenBall);
3markerListRenderSettings.highDensityPointsGroupIcon = ServicesManager.createIconFromId(Icon.BlueBall);
How it works
In Qt, go to the File menu and select Open File or Project…
then browse to the MarkersProgrammatic example folder and open MarkersProgrammatic.pro
You may want to have a look at Setting your API Key to see how to open and configure a project and set your API Key.
Sketches-type markers¶
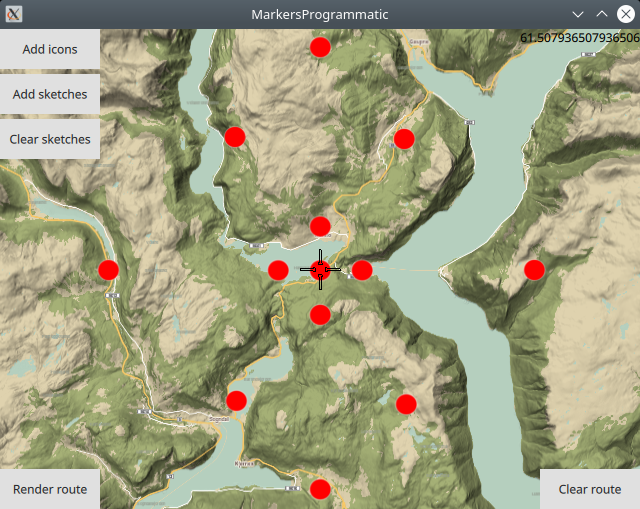
Sketches-type markers cannot be grouped. The following function shows how a sketches-type marker is created, given its latitude and longitude coordinates on the map, in degrees (as double values).
1function createSketchesPoi(lat, lon)//sketches-type markers - cannot be grouped;
2{
3 let coords = ServicesManager.createCoordinates(lat,lon,0);//lat,lon,alt
4
5 /////////////////////////////////
6 //the following optional block is used only to enable autocenter on landmark group
7 //and to render a route between the individual landmarks/waypoints/icons;
8 {
9 //Creates a landmark that is owned by the routingWaypoints, for efficiency.
10 //This is required because
11 //routingWaypoints.append(lmk);
12 //will clone the landmark unless the owner is the routingWaypoints list itself.
13 let lmk = ServicesManager.createLandmark(routingWaypoints);
14 lmk.coordinates = coords;
15 routingWaypoints.append(lmk);
16 }
17 /////////////////////////////////
18
19 return coords;
20}
Note that the createSketchesPoi()
function contains an optional
block of code which is used to fly the camera to the location of the
group of markers, such that the entire group (the bounding box
containing the list of specified markers) fits in the viewport.
This optional functionality requires that the coordinates for each
marker is also added to a separate list: routingWaypoints.append(lmk);
This separate list, routingWaypoints
, can also be used to
optionally render a route between the markers, using them as
waypoints, in the order in which they were added to the list.
1Button {
2 text: "Add sketches"
3 onClicked: {
4 let marker = ServicesManager.createMarker();
5 let markerRenderSettings = ServicesManager.createMarkerRenderSettings();
6
7 markerRenderSettings.imageSize = 32;
8 markerRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/redcircle.png")
9 //markerRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/greensquare.png")
10 //markerRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/bluetriangle.png")
11
12 //if the list is not cleared, it will grow every time the button is clicked;
13 routingWaypoints.clear();//clear the list before adding points;
14
15 //sketches-type markers - cannot be grouped;
16 //latitude and longitude of each icon to be added to the map
17 marker.append(createSketchesPoi(61.3,7.16));//lat,lon
18 marker.append(createSketchesPoi(61.3,7.2));//lat,lon
19 marker.append(createSketchesPoi(61.3,7.24));//lat,lon
20 marker.append(createSketchesPoi(61.3,7.4));//lat,lon
21 marker.append(createSketchesPoi(61.3,7.0));//lat,lon
22 marker.append(createSketchesPoi(61.2,7.2));//lat,lon
23 marker.append(createSketchesPoi(61.4,7.2));//lat,lon
24 marker.append(createSketchesPoi(61.28,7.2));//lat,lon
25 marker.append(createSketchesPoi(61.32,7.2));//lat,lon
26 marker.append(createSketchesPoi(61.24,7.12));//lat,lon
27 marker.append(createSketchesPoi(61.24,7.28));//lat,lon
28 marker.append(createSketchesPoi(61.36,7.12));//lat,lon
29 marker.append(createSketchesPoi(61.36,7.28));//lat,lon
30
31 let sketchlist = map.markerCollection.getExtendedList(MarkerList.Type.Point);
32 sketchlist.append(marker, markerRenderSettings);
33
34 //the following block is used only to autocenter on the group of landmarks;
35 {
36 map.centerOnGeographicArea(routingWaypoints.boundingBox)
37 }
38 }
39}
The Add sketches
button contains the code to add the
sketches-type markers to the map, which cannot be grouped upon zooming.
First, the sketches-type marker
and its
corresponding markerRenderSettings
are instantiated.
Next, a custom icon
image is set, along with its imageSize
in pixels.
The custom icon image to represent the markers on the map can
be replaced by one of the other configured images, by uncommenting
the line with the green or blue png file instead.
The image filename is configured in qml.qrc
and the png image itself
is located in the project directory.
Then the latitude, longitude coordinates of each sketches-type marker is added to the list.
1let sketchlist = map.markerCollection.getExtendedList(MarkerList.Type.Point);
2sketchlist.append(marker, markerRenderSettings);
Next, a pointer to the built-in sketches-type marker list is obtained, and then the list of sketches-type markers, and their corresponding settings, including icon image and size, are added, to be displayed on the map.
Rendering a route¶
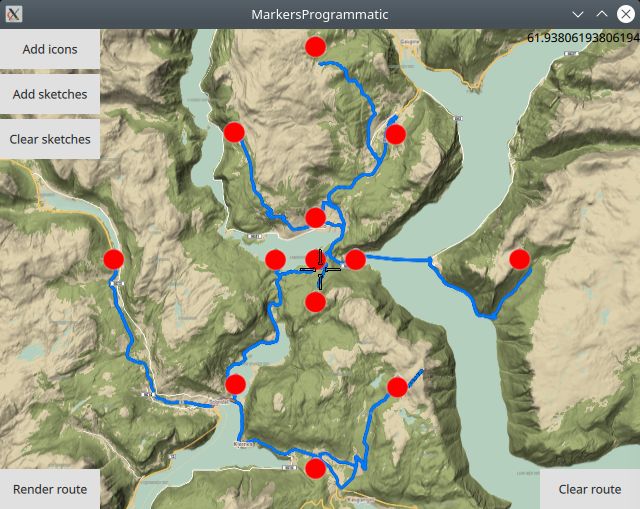
Optionally, a route can be rendered using the markers as waypoints, in the order in which they were added.
1RoutingService {
2 id: routingService
3 type: Route.Type.Fastest
4 transportMode: Route.TransportMode.Car
5 // The list of waypoints is made available to the routing service.
6 waypoints: routingWaypoints
7 onFinished: {
8 map.routeCollection.set(routeList);
9 map.centerOnRouteList(routeList);
10 }
11}
A RoutingService is defined, used in this example only to
compute and render a route plotted using the list of waypoints/landmarks
in the order in which they were added to the list;
this block is not required to autocenter on the landmark group.
Set internet connection to true in the Component.onCompleted
block
for route computation and rendering to work.
Removing sketches-type markers¶
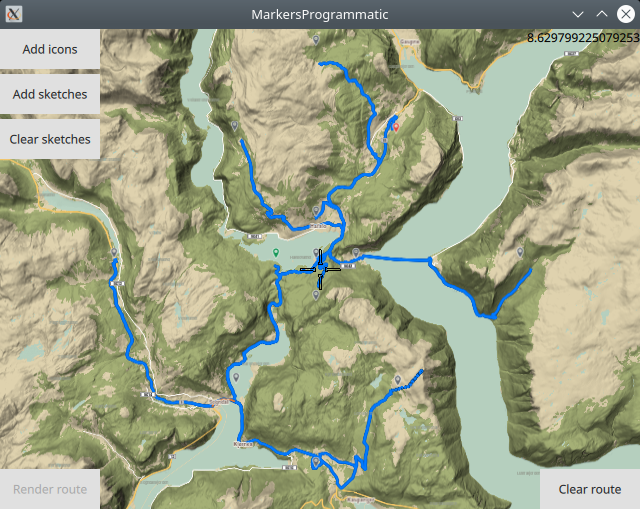
The route and/or sketches-type markers can be removed from the map separately.
1Button {
2 text: "Clear sketches"
3 onClicked: {
4 routingWaypoints.clear();
5 let sketchlist = map.markerCollection.getExtendedList(MarkerList.Type.Point);
6 sketchlist.clear();
7 }
8}
Removing the sketches-type markers is done by again getting a pointer
to the built-in sketches-type marker list, and using the clear()
function.
The corresponding routingWaypoints
list, used to render the
route or center on the group of markers, or both, is also cleared.
The same set of coordinates is in each of these lists,
so both must be cleared.
Regular markers¶
Regular markers can be grouped. The following function shows how a regular marker is created, given its latitude and longitude coordinates on the map, in degrees (as double values).
1function createMarkerPoi(lat, lon)//regular markers - can be grouped;
2{
3 let coords = ServicesManager.createCoordinates(lat,lon,0);//lat,lon,alt
4 let marker = ServicesManager.createMarker();
5 marker.append(coords);
6
7 /////////////////////////////////
8 //the following optional block is used only to enable autocenter on landmark group
9 //and to render a route between the individual landmarks/waypoints/icons;
10 {
11 //Creates a landmark that is owned by the routingWaypoints, for efficiency.
12 //This is required because
13 //routingWaypoints.append(lmk);
14 //will clone the landmark unless the owner is the routingWaypoints list itself.
15 let lmk = ServicesManager.createLandmark(routingWaypoints);
16 lmk.coordinates = coords;
17 routingWaypoints.append(lmk);
18 }
19 /////////////////////////////////
20
21 return marker;
22}
The createMarkerPoi()
function contains an optional
block of code which is used to fly the camera to the location of the
group of markers, such that the entire group (the bounding box
containing the list of specified markers) fits in the viewport.
This optional functionality requires that the coordinates for each
marker is also added to a separate list: routingWaypoints.append(lmk);
This separate list, routingWaypoints
, can also be used to
optionally render a route between the markers, using them as
waypoints, in the order in which they were added to the list.
1text: map.markerCollection.listCount>0
2? qsTr("Clear icons"): qsTr("Add icons")
The Add icons
/Clear icons
button has functionality to both
add and remove the regular markers, which are added as a MarkerList
to the built-in map.markerCollection
and thus, if this collection
is not empty, then a regular marker list was previously added, so the
Clear icons
remove regular markers functionality is active.
The Add icons
block to add regular markers which can be
grouped upon zooming, is shown here:
First, the regular markerList
and its
corresponding markerListRenderSettings
are instantiated.
Next, a custom icon
image is set, along with its imageSize
in pixels.
This image is used to represent single markers.
The specified labeling mode indicates that when icons are grouped, a number
is rendered under the group, to indicate the number of icons in the group.
Zoom level 98 is near the surface.
1{
2 //regular markers - can be grouped;
3 let markerlist = ServicesManager.createMarkerList(MarkerList.Type.Point);
4 let markerListRenderSettings = ServicesManager.createMarkerListRenderSettings();
5
6 markerListRenderSettings.imageSize = 32;
7 markerListRenderSettings.icon = ServicesManager.createIconFromFile("qrc:/violetcircle.png");
8 markerListRenderSettings.labelingMode = MarkerRenderSettings.Group;
9
10 markerListRenderSettings.pointsGroupingZoomLevel = 98;
11 markerListRenderSettings.lowDensityPointsGroupMaxCount = 6;
12 markerListRenderSettings.lowDensityPointsGroupIcon = ServicesManager.createIconFromFile("qrc:/redcircle.png");
13 //markerListRenderSettings.lowDensityPointsGroupIcon = ServicesManager.createIconFromId(Icon.RedBall);
14 markerListRenderSettings.mediumDensityPointsGroupMaxCount = 9;
15 markerListRenderSettings.mediumDensityPointsGroupIcon = ServicesManager.createIconFromFile("qrc:/greensquare.png");
16 //markerListRenderSettings.mediumDensityPointsGroupIcon = ServicesManager.createIconFromId(Icon.GreenBall);
17 markerListRenderSettings.highDensityPointsGroupMaxCount = 12;
18 markerListRenderSettings.highDensityPointsGroupIcon = ServicesManager.createIconFromFile("qrc:/bluetriangle.png");
19 //markerListRenderSettings.highDensityPointsGroupIcon = ServicesManager.createIconFromId(Icon.BlueBall);
20
21 //set a map style configured in qml.qrc, and located in the project directory;
22 //choose one map style line and uncomment it;
23 //map.stylePath = "qrc:/Basic_1_Night_Blues_with_Elevation-1_5_688.style";
24 map.stylePath = "qrc:/Basic_1_Oldtime_with_Elevation-1_12_657.style";
25
26 //if the list is not cleared, it will grow every time the button is clicked;
27 routingWaypoints.clear();//clear the list before adding points;
28
29 //regular markers - can be grouped;
30 //latitude and longitude of each icon to be added to the map
31 markerlist.append(createMarkerPoi(61.3,8.66));//lat,lon
32 markerlist.append(createMarkerPoi(61.3,8.7));//lat,lon
33 markerlist.append(createMarkerPoi(61.3,8.74));//lat,lon
34 markerlist.append(createMarkerPoi(61.3,8.9));//lat,lon
35 markerlist.append(createMarkerPoi(61.3,8.5));//lat,lon
36 markerlist.append(createMarkerPoi(61.2,8.7));//lat,lon
37 markerlist.append(createMarkerPoi(61.4,8.7));//lat,lon
38 markerlist.append(createMarkerPoi(61.28,8.7));//lat,lon
39 markerlist.append(createMarkerPoi(61.32,8.7));//lat,lon
40 markerlist.append(createMarkerPoi(61.24,8.62));//lat,lon
41 markerlist.append(createMarkerPoi(61.24,8.78));//lat,lon
42 markerlist.append(createMarkerPoi(61.36,8.62));//lat,lon
43 markerlist.append(createMarkerPoi(61.36,8.78));//lat,lon
44
45 //MARKERS
46 map.markerCollection.appendList(markerlist, markerListRenderSettings);
47
48 //the following block is used only to autocenter on the group of landmarks;
49 {
50 map.centerOnGeographicArea(routingWaypoints.boundingBox)
51 }
52}
Three additional icon images are configured, one for each of the low-,
medium- and high- density levels of marker icon grouping.
In this case, lowDensityPointsGroupMaxCount = 6
means that the configured
lowDensityPointsGroupIcon
is rendered for groups of more than 1
but less than 6 marker icons.
Similarly, mediumDensityPointsGroupMaxCount = 9
means that the
mediumDensityPointsGroupIcon
is rendered for groups of
equal to or more than 6 but less than 9 marker icons.
Groups of 9 or more icons will be rendered using the highDensityPointsGroupIcon
.
The image filenames are configured in the qml.qrc
file which is located
in the project directory along with the png images.
Alternatively, it is possible to use the preconfigured icons by uncommenting
the lines with Icon.RedBall
, Icon.GreenBall
and Icon.BlueBall
enums respectively.
Next, a map style is set, which is also located in the project
directory and configured in the qml.qrc file.
The routingWaypoints
list is cleared/emptied before adding
the marker coordinates, to keep it from growing, if the button is
clicked multiple times.
This list is not used for rendering marker icons on the map, only
for centering the area containing the marker icons in the viewport,
and optionally rendering a route through the markers as waypoints.
Then the latitude, longitude coordinates of each marker is added to the markerlist.
map.markerCollection.appendList(markerlist, markerListRenderSettings);
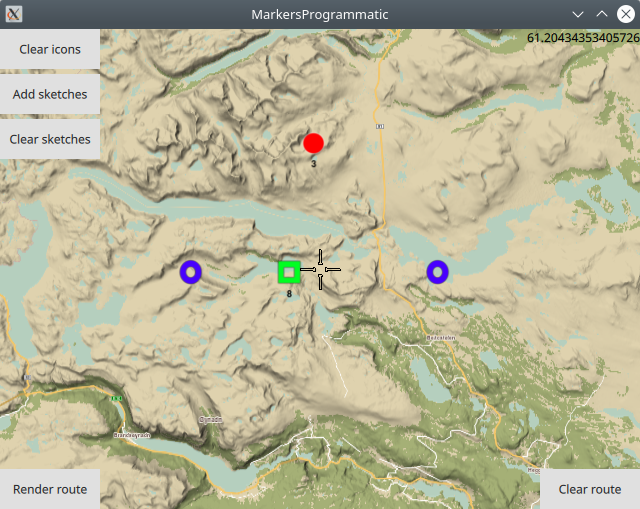
Finally, the list of markers and its corresponding settings is
added to the built-in map.markerCollection
to be rendered
on the map.
In this image, the purple circles are single markers,
the red icons are low density groups (more than 1 but less than 6),
and the green icons are medium density groups (greater than or equal to 6).
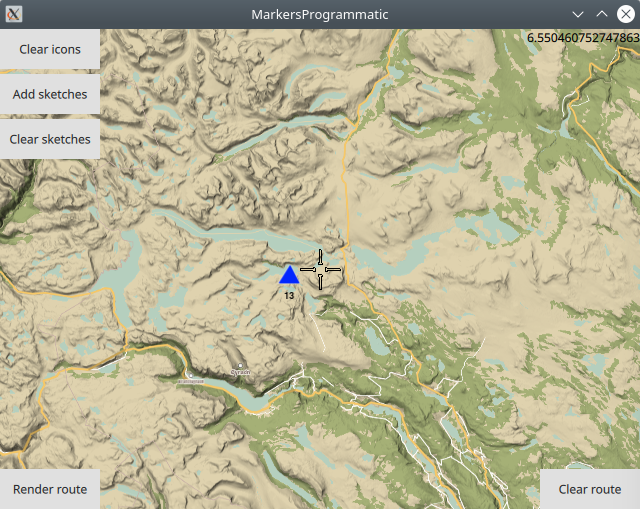
In this image, the blue triangle is a high density group (9 or more markers).
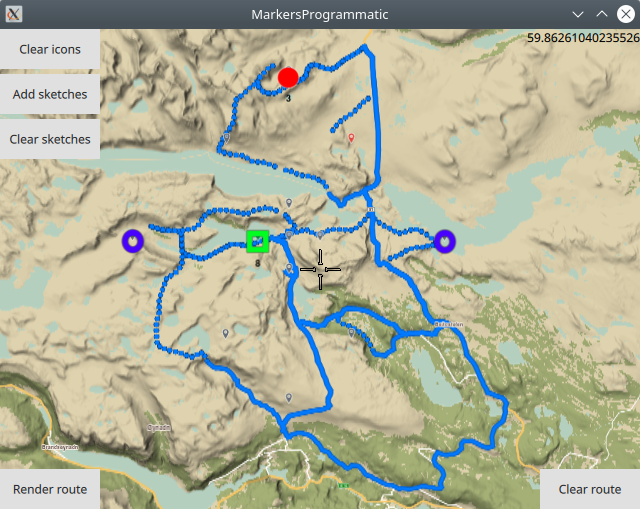
A route can be rendered using the markers as waypoints, the same as for the sketches-type markers. Even if some marker icons are grouped, the route is computed through all markers, in the order in which they were added.
Removing markers¶
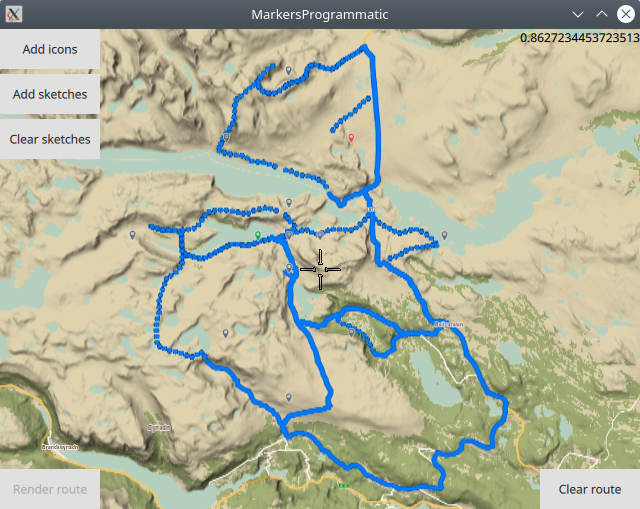
The icons and route can be removed from the map separately. In this case, the marker icons were removed.
1Button {
2 text: map.markerCollection.listCount>0
3 ? qsTr("Clear icons"): qsTr("Add icons")
4 onClicked: {
5 if (map.markerCollection.listCount>0)
6 {
7 //show how to clear icons from the map;
8 routingWaypoints.clear();
9 map.markerCollection.removeList(0);//markers
10 map.update();
11 }
12 else
13 {
14 ...
15 }
16 }
17}
If the built-in if (map.markerCollection.listCount>0)
is not empty, that is, the listCount is greater than 0, that
means a marker list was previously added, so the Clear icons
block is active for the button that either adds/removes the
regular markers to/from the map.
The marker list containing the coordinates for all regular marker
icons is in the built-in list at index 0, as it is the first object
added to the list, so to clear the regular markers from the map,
remove the object at index 0, then redraw/refresh the map, which
causes the icons to disappear from the map.
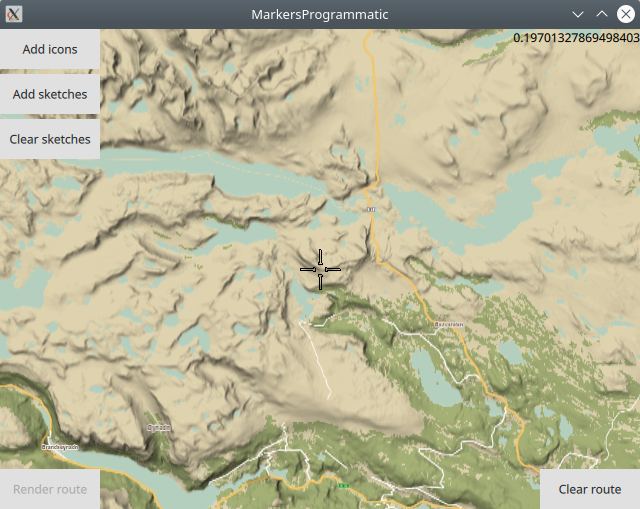
Also clear the routingWaypoints used for centering on the bounding box containing the markers, as the same set of coordinates is in each of these 2 lists, so both must be cleared.